Writing Your First Playwright Test Script
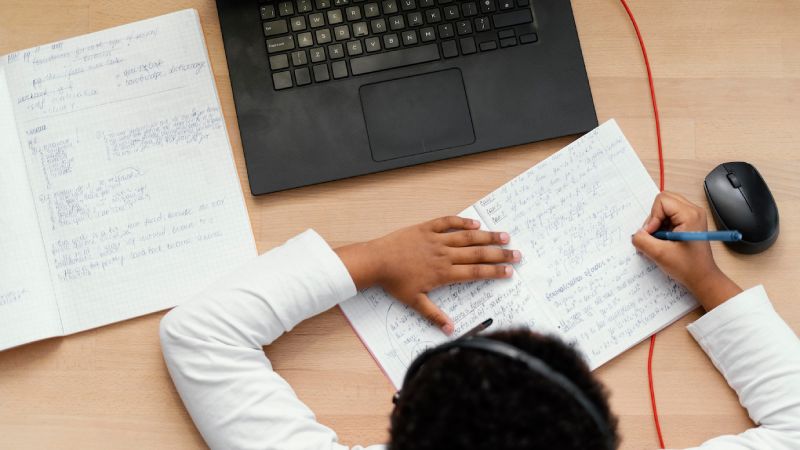
In the rapidly evolving landscape of web development, ensuring that your web applications are robust, secure, and user-friendly is non-negotiable. One tool that is increasingly gaining traction to help accomplish this is Playwright. An open-source Node.js library, Playwright enables developers to write high-quality end-to-end tests in modern web browsers. It is versatile, capable, and quite powerful when it comes to automating challenging scenarios.
Why are test scripts important, you ask? They’re the building blocks that assure developers and stakeholders that changes in code—whether they’re feature implementations or bug fixes—have not disrupted the existing functionalities. As developers continue to prioritize building dynamic and complex applications, the role of automation testing tools like Playwright has become crucial.
In this blog post, we will guide you through the A to Z of writing your first Playwright test script. We’ll start by setting up your environment, then move to writing basic to advanced test scripts, and finally wrap up with how you can leverage LambdaTest to execute cross-browser tests effortlessly.
Getting Started with Playwright
Defining Playwright and Its Key Features
Playwright is a Node.js library aimed at automating web browsers to facilitate various kinds of browser automation tasks, including but not limited to end-to-end testing, web scraping, and monitoring web pages over time. It was created to overcome the limitations of existing testing libraries, offering a more reliable, efficient, and comprehensive toolset.
Here are some key features of Playwright that make it a go-to tool for web testing:
- Multiple Browser Support: Playwright supports multiple browsers, including Chromium, Firefox, WebKit, and Microsoft’s Edge, offering a cross-browser testing environment out of the box.
- Mobile Viewports: Playwright allows you to emulate multiple viewport sizes and screen orientations, facilitating mobile web testing.
- Intercept Network Activity: It gives you the ability to observe, modify, and log the network activity during your tests, making it possible to test the application’s response to various server conditions.
- Rich Input Emulation: From keyboard inputs to touchscreen gestures, Playwright can emulate a plethora of user interactions.
- Capture Screenshots and Videos: Playwright can capture screenshots and videos, making it easier to debug and understand test behavior.
- Headless Browsing: Running browsers in headless mode makes it ideal for integrating into CI/CD pipelines.
- Concurrency: It supports parallel test execution, thereby reducing the total test execution time.
Supported Browsers and Platforms
Playwright is designed to support a variety of web browsers:
- Chromium: The open-source web browser project that forms the basis for Google Chrome.
- Firefox: Mozilla’s web browser known for its focus on speed and privacy.
- WebKit: The engine behind Safari, primarily used on macOS and iOS devices.
- Microsoft Edge: Microsoft’s Chromium-based browser.
Furthermore, Playwright is compatible with Windows, macOS, and Linux, providing a versatile testing environment.
Setting Up the Development Environment
Pre-requisites:
Before diving into Playwright, you’ll need to have Node.js and npm (Node Package Manager) installed. If you don’t have these, you can download and install them from the Node.js official website.
Step-by-step Instructions to Install Playwright:
- Open Terminal and Create a New Directory:
mkdir my-playwright-project
cd my-playwright-project
- Initialize a New Node.js Project:
npm init -y
This command creates a package.json file for your project.
- Install Playwright:
npm install playwright
This command installs Playwright and its dependencies. It also downloads browser binaries for Chromium, Firefox, and WebKit.
And voila! You’ve successfully set up a development environment to write and execute Playwright tests.
Writing Your First Playwright Test
Basic Structure of a Playwright Test Script
When creating a test script in Playwright, you usually start by importing the Playwright library, followed by the instantiation of a browser instance. Once you have a browser instance, you can then open a new page and perform actions like clicks, input typing, and form submissions.
Here’s a rudimentary rundown of what the sequence often looks like:
- Import Playwright
- Initialize a browser instance
- Open a new page in the browser
- Perform actions on the page
- Assertion and Validation
- Close the browser
Creating a New Test File
- Create a new file in your project folder, for instance, myFirstTest.js.
Step-by-step Code Walkthrough
Import Playwright
The first step is to import the Playwright library:
const { chromium } = require(‘playwright’);
Initialize a Browser Instance
Now, let’s launch a browser. Since we’ve imported Chromium, we will initialize a new Chromium browser instance.
const browser = await chromium.launch();
Open a New Page
After launching the browser, the next step is to open a new page:
const page = await browser.newPage();
Perform Actions on the Page
Let’s navigate to a website and interact with it. For instance, going to the Bing search engine homepage and typing ‘Playwright’ in the search bar:
await page.goto(‘https://www.bing.com’);
await page.type(‘[aria-label=”Enter your search term”]’, ‘Playwright’);
await page.press(‘[aria-label=”Enter your search term”]’, ‘Enter’);
Assertion and Validation
To validate that the search worked correctly, you could check the title of the resulting page:
const title = await page.title();
console.assert(title === ‘Playwright – Bing’);
Close the Browser
Finally, don’t forget to close the browser.
await browser.close();
Code Example
Combining all these steps, your complete test script could look like this:
const { chromium } = require(‘playwright’);
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto(‘https://www.bing.com’);
await page.type(‘[aria-label=”Enter your search term”]’, ‘Playwright’);
await page.press(‘[aria-label=”Enter your search term”]’, ‘Enter’);
const title = await page.title();
console.assert(title === ‘Playwright – Bing’);
await browser.close();
})();
Importance of Organization
Code readability and maintainability are critical in software development. It’s good to give your tests descriptive names and use comments where necessary. Consider separating your tests into different files based on functionalities or modules they are testing. This makes it easier for other developers or even future you to understand what each test does.
Advanced Playwright Techniques
Handling Authentication, iframes, and Pop-ups
Playwright offers multiple ways to handle scenarios like authentication, iframes, and pop-up dialogs. For instance, you can navigate to a login page, enter credentials, and click the ‘Submit’ button to authenticate a user session.
To interact with iframes, you can use methods like page.frame to obtain a frame object, and then you can interact with this object similar to how you interact with a page.
Handling pop-up dialogs like alerts, prompts, and confirms is straightforward using Playwright’s dialog event.
Waiting for Elements
It’s often necessary to wait for elements to appear or load on the page. Playwright provides several waiting methods, such as waitForSelector and waitForTimeout, which allow you to pause script execution until a particular condition is met.
await page.waitForSelector(‘#elementID’);
Simulating User Interactions
Playwright provides an API to simulate complex user interactions like drag-and-drop, clicking, typing, and even touch events for mobile web testing.
await page.dragAndDrop(‘#source’, ‘#target’);
await page.click(‘#buttonID’);
await page.type(‘#inputID’, ‘Type this text’);
This wraps up Chapter 3, which should equip you with advanced techniques to create more versatile and complex test scripts.
Running Playwright Tests Locally
Running Your Tests
Once you’ve written your Playwright tests, you will want to execute them to see the results. Running tests locally is quite straightforward. Navigate to your project directory in the terminal and execute the test file using Node.js:
node myFirstTest.js
This command will run your test and you should see Chromium automating the tasks you scripted, such as opening the browser, navigating to the page, and performing actions.
Benefits of Local Testing
Local testing is a vital part of the development cycle for several reasons:
- Debugging: Running tests locally makes it easier to debug issues in real-time. You can also use debugging tools and logs to trace problems.
- Speed: Local tests generally execute faster since they don’t have to deal with network latency.
- Flexibility: Local testing allows you to run tests during development iteratively, making adjustments to the code as necessary.
- Cost-Effectiveness: It doesn’t require an internet connection or cloud compute resources, potentially saving costs.
Interpreting Test Results
Understanding test results is crucial for improving code quality. Playwright’s API can be extended to include custom loggers or integrate with test result visualization tools, providing insights into test failures or bugs.
Leveraging LambdaTest for Cross Browser Testing with Playwright
LambdaTest is an AI-powered test orchestration and execution platform that empowers devs and testers to perform Playwright testing across 40+ real browsers and OS combinations.
This chapter will guide you through the process of setting up, running your first test, and viewing the results on LambdaTest using Playwright.
Setting Up LambdaTest with Playwright
Prerequisites:
Before running your tests on LambdaTest, ensure that you have Playwright installed. You can run tests using Playwright versions from v1.15.0 to the latest.
Steps:
1. Clone the LambdaTest-Playwright Repository:
First, clone the LambdaTest-Playwright repository onto your system. All the code samples for this documentation can be found there.
git clone <LambdaTest-Playwright repository URL>
2. Install Dependencies:
Navigate to the cloned repository folder and run:
npm install
3. Set Environment Variables:
You need to set your LambdaTest username and access key as environment variables. These can be found in your LambdaTest dashboard.
For Windows:
set LT_USERNAME=”YOUR_LAMBDATEST_USERNAME”
set LT_ACCESS_KEY=”YOUR_LAMBDATEST_ACCESS_KEY”
For macOS/Linux:
export LT_USERNAME=”YOUR_LAMBDATEST_USERNAME”
export LT_ACCESS_KEY=”YOUR_LAMBDATEST_ACCESS_KEY”
4. Update the Browser WebSocket Endpoint:
Add the following line to your test script to specify the LambdaTest remote grid URL:
wsEndpoint: `wss://cdp.lambdatest.com/playwright?capabilities=${encodeURIComponent(JSON.stringify(capabilities))}`
Running Your First Test on LambdaTest
Once you’ve set up your environment, running your first test is the next milestone. Here’s how to run a basic test that searches for the term “LambdaTest” on Bing.
First, add the capabilities and WebSocket Endpoint in your script as follows:
const { chromium } = require(‘playwright’);
(async () => {
const capabilities = {
‘browserName’: ‘Chrome’,
‘browserVersion’: ‘latest’,
‘LT:Options’: {
‘platform’: ‘Windows 10’,
‘build’: ‘Playwright Sample Build’,
‘name’: ‘Playwright Sample Test’,
‘user’: process.env.LT_USERNAME,
‘accessKey’: process.env.LT_ACCESS_KEY,
‘network’: true,
‘video’: true,
‘console’: true
}
}
const browser = await chromium.connect({
wsEndpoint: `wss://cdp.lambdatest.com/playwright?capabilities=${encodeURIComponent(JSON.stringify(capabilities))}`
});
// … (rest of your test script)
})();
To run the test, navigate to your project directory and execute:
node playwright-single.js
Viewing Your Test Results on LambdaTest
After your test has completed, you can view the results in the LambdaTest Automation Dashboard. The dashboard displays an array of information:
- Test Builds: You can see a list of test builds on the left side of the dashboard.
- Build Sessions: Upon clicking a test build, you can see the individual build sessions related to that build on the right side.
- Test Details: Clicking on the session name opens a detailed view of the test execution, including selected configurations, logs, and videos.
- Assertions and Status: LambdaTest allows you to mark your tests as passed or failed based on assertions, which is also displayed in the dashboard.
- Screenshots and Video Logs: For more in-depth analysis, LambdaTest provides screenshots and video logs of your test execution.
- Additional Information: You can find more information like Test ID, Test Name, and other metadata, helping you in debugging and documentation.
By using Playwright and LambdaTest together, you can not only run your browser tests efficiently but also ensure that your web application is compatible across different browsers and platforms. With detailed test results, debugging becomes easier, enhancing your overall testing experience.
Analyzing Test Results
After running tests on LambdaTest, you can view comprehensive test results on their dashboard. LambdaTest provides capabilities like screenshots, video recording, and logs to help analyze test runs. This helps not just in debugging but also in understanding how the application behaves under different scenarios.
Conclusion
In the fast-paced world of web development, tools like Playwright have emerged as lifesavers, offering robust, reliable, and scalable testing solutions. Coupled with cloud-based platforms like LambdaTest, they provide an exhaustive environment for ensuring that your web applications run flawlessly across different browsers and platforms.
Whether you are new to browser automation and testing, or an experienced developer looking to switch to a more powerful tool, Playwright, with its plethora of features and capabilities, is worth exploring.